t1 table
+----+--------+-------+
| id | name | age |
+-------------+-------+
| 1 | kusal | 25 |
| 2 | saman | 20 |
+----------+----+-----+
t2 table
+----------+----+------------+
| image_id | id | image_path |
+----------+----+------------+
| 1 | 1 | path1 |
| 2 | 1 | path2 |
| 3 | 1 | path 3 |
| 4 | 2 | path 1 |
| 5 | 1 | path 4 |
| 6 | 2 | path 5 |
+----------+----+------------+
SELECT * FROM t1 a left join t2 b on a.id = b.id group by b.id
+----+-------+-----+----------+------+------------+
| id | name | age | image_id | id | image_path |
+----+-------+-----+----------+------+------------+
| 1 | kusal | 25 | 1 | 1 | path1 |
| 2 | saman | 21 | 4 | 2 | path 1 |
+----+-------+-----+----------+------+------------+
Using group by for the right table id, it will only get the one result from right table.
Monday, November 16, 2009
Tuesday, October 27, 2009
Drop Up Menu - AdxMenu
Here is a cool Drop up menu you can use in your site.
http://aplus.rs/adxmenu/examples/hbt/
http://aplus.rs/adxmenu/examples/
Friday, June 26, 2009
Fix - Facebook profile application tab blank page
I think many new Facebook application developers face this problem. You do all the steps instructed in a book or the documentation and you still get a blank page for the profile tab. Yeah I know the feeling, It sucks big time, This error almost made me stop learning about Facebook app development.
After hours of searching and experimenting I found the solution for my problem.
Solution for my problem:
Canvas Callback URL should have a trailing /
Ex:
Canvas Callback URL: http://xxxxxx.joyent.us/
If you are using http://yourdomain/index.php just remove index.php since it will be loaded in default.
After hours of searching and experimenting I found the solution for my problem.
Solution for my problem:
Canvas Callback URL should have a trailing /
Ex:
Canvas Callback URL: http://xxxxxx.joyent.us/
If you are using http://yourdomain/index.php just remove index.php since it will be loaded in default.
Wednesday, June 17, 2009
SEO Title Tag - 63 Characters or Low
In my opinion html title tag is most important thing in SEO optimization.
Creating meaningful and keyword specific titles is a must if you need a high rank in search engines.
Things to consider when writing web page titles
Creating meaningful and keyword specific titles is a must if you need a high rank in search engines.
Things to consider when writing web page titles
- Keep the title less than 63 (max: 70) characters.
- Put the important keywords in the front as possible.
- Putting the site name in the tilte is a choice you have to make with lot of thought. If you think that the site name has a good branding effect you can put the name at the end or front.
- Without using and, or, is try to use comma or |
- Don't use any meaningless words or special characters
- Never use duplicate titles on pages
Sunday, June 7, 2009
How to fix You don't have permission to access /phpmyadmin/ on this server Wamp
You don't have permission to access /phpmyadmin/ on this server (WAMP)
This is the message I got when I tried to access phpmyadmin after reinstalling WAMP server to a newer version. After some searching I was able to find the problem.
Reason:
When you uninstall your previous WAMP server there will be still few files that will be left not deleted and will not be replaced by the new install. One such file is the phpmyadmin.conf file that is under C:\wamp\alias folder. This file holds the actual path to the phpmyadmin folder inside WAMP.
If the new WAMP server has a new phpmyadmin version this phpmyadmin.conf will still point to the old folder which is not existing inside WAMP.
Solution:
goto C:\wamp\alias\ and edit the phpmyadmin.conf file using notepad
I just edited the c:/wamp/apps/phpmyadmin2.11.6/ with c:/wamp/apps/phpmyadmin3.1.3.1/
To find your new phpmyadmin folder goto C:\wamp\apps\
Now restart the WAMP server and try to access phpmyadmin
This is the message I got when I tried to access phpmyadmin after reinstalling WAMP server to a newer version. After some searching I was able to find the problem.
Reason:
When you uninstall your previous WAMP server there will be still few files that will be left not deleted and will not be replaced by the new install. One such file is the phpmyadmin.conf file that is under C:\wamp\alias folder. This file holds the actual path to the phpmyadmin folder inside WAMP.
If the new WAMP server has a new phpmyadmin version this phpmyadmin.conf will still point to the old folder which is not existing inside WAMP.
Solution:
goto C:\wamp\alias\ and edit the phpmyadmin.conf file using notepad
Alias /phpmyadmin "c:/wamp/apps/phpmyadmin2.11.6/"
# to give access to phpmyadmin from outside
# replace the lines
#
# Order Deny,Allow
# Deny from all
# Allow from 127.0.0.1
#
# by
#
# Order Allow,Deny
# Allow from all
#
<Directory "c:/wamp/apps/phpmyadmin2.11.6/">
Options Indexes FollowSymLinks MultiViews
AllowOverride all
Order Deny,Allow
Deny from all
Allow from 127.0.0.1
</Directory>
I just edited the c:/wamp/apps/phpmyadmin2.11.6/ with c:/wamp/apps/phpmyadmin3.1.3.1/
To find your new phpmyadmin folder goto C:\wamp\apps\
Alias /phpmyadmin "c:/wamp/apps/phpmyadmin3.1.3.1/"
# to give access to phpmyadmin from outside
# replace the lines
#
# Order Deny,Allow
# Deny from all
# Allow from 127.0.0.1
#
# by
#
# Order Allow,Deny
# Allow from all
#
<Directory "c:/wamp/apps/phpmyadmin3.1.3.1/">
Options Indexes FollowSymLinks MultiViews
AllowOverride all
Order Deny,Allow
Deny from all
Allow from 127.0.0.1
</Directory>
Now restart the WAMP server and try to access phpmyadmin
Saturday, June 6, 2009
Best Free php and Mysql web hosting with cPanel
000webhost offer completely ad-free cPanel web hosting.
No ads will ever be forced onto our users webpages.
There are no catches.
No setup fees.
No forced advertising.
No banners.
No popups
No hidden charges.
Only totally free hosting service.
Register now, here is what waiting for you:
* 1500 MB Disk Space
* 100 GB Data Transfer
* cPanel Control Panel
* Website Builder
* 5 MySQL Databases
* Unrestricted PHP5 support
* Instant Setup!
This service is perfect for starting a new online community, blog or personal website! More info at http://www.000webhost.com/171354.html
No ads will ever be forced onto our users webpages.
There are no catches.
No setup fees.
No forced advertising.
No banners.
No popups
No hidden charges.
Only totally free hosting service.
Register now, here is what waiting for you:
* 1500 MB Disk Space
* 100 GB Data Transfer
* cPanel Control Panel
* Website Builder
* 5 MySQL Databases
* Unrestricted PHP5 support
* Instant Setup!
This service is perfect for starting a new online community, blog or personal website! More info at http://www.000webhost.com/171354.html
Tuesday, May 19, 2009
Create Dynamic Images with Text Using PHP GD
First check weather php gd library is enabled.
If you get a error or a blank page that means the gd is not enabled in your server.
Contact your hosting and ask for php gd to be enabled.
Try following coding, everything is commented.
You can use this php file in a html file like this also
<?PHP
echo '<pre>';
print_r(gd_info());
echo '</pre>';
?>
If you get a error or a blank page that means the gd is not enabled in your server.
Contact your hosting and ask for php gd to be enabled.
Try following coding, everything is commented.
<?PHP
//save the arial.ttf font file in the same folder
$font = 'arial.ttf';
$fontsize = 10;
$YourText = 'Hi This Work';
$Text_Box = imagettfbbox($fontsize, 0, $font, $YourText);
//create image width 250, height 100
$image = imagecreatetruecolor(250,100);
//0,0,0 is black
$bgcolor = imagecolorallocate($image, 0, 0, 0);
//255, 255, 255 is white
$fontcolor = imagecolorallocate($image, 255, 255, 255);
//create a rectangle with the back ground coloe
imagefilledrectangle($image, 0, 0, 250, 100, $bgcolor);
$x = 10;
$y = 20;
//draw text on image
imagettftext($image, $fontsize, 0, $x, $y, $fontcolor, $font, $YourText);
//setting png headers
header('Content-type: image/png');
//create png image
imagepng($image);
//delete tempory files
imagedestroy($image);
?>
You can use this php file in a html file like this also
<img src="your_file_name.php" />
Sunday, May 17, 2009
phpMyAdmin mysql table overhead
If you are using phpmyadmin to manage mysql databases and tables you have probably seen a red figure for overhead in the space usage section in a table's detailed view.
This is a usual thing when you have lot of deletes and updates in your table fields. Think overhead as empty space and mysql want it to be defragmented. You can simply use the Optimize table link to get rid of the overhead.
Thursday, May 14, 2009
Create your own Konami code or custom code.
I have used Paul Irish code for my example.
By replacing unicode char values in this line you can define any key combination you like.
Example
var kkeys = [], konami = "38,40,75";
this means up,down,k
You can find any key code from this site http://www.javascriptkit.com/jsref/eventkeyboardmouse.shtml
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.3.2/jquery.min.js"></script>
<script>
jQuery(function($){
var kkeys = [], konami = "38,38,40,40,37,39,37,39,66,65";
$(document).keydown(function(e)
{
kkeys.push( e.keyCode );
if( kkeys.toString().indexOf( konami ) >= 0 )
{
$(document).unbind('keydown',arguments.callee);
//Any message or event you want goes here
alert('The Konami Code Is Activated');
}
});
});
</script>
By replacing unicode char values in this line you can define any key combination you like.
Example
var kkeys = [], konami = "38,40,75";
this means up,down,k
You can find any key code from this site http://www.javascriptkit.com/jsref/eventkeyboardmouse.shtml
Tuesday, May 12, 2009
Use Gmail to send mails in php
You can use Gmail SMTP to send emails remotely even in your localhost machine with WAMP.
First you must download phpmailer
After you extratced all zip files goto examples folder. In there you will find test_smtp_gmail_basic.php file. Just edit the code with your gmail username and password and you are ready to go.
Before executing the script goto php.ini file and remove the ; that is infront of extension=php_openssl.dll
Then restart your server.
Leave a comment if you need any help.
First you must download phpmailer
After you extratced all zip files goto examples folder. In there you will find test_smtp_gmail_basic.php file. Just edit the code with your gmail username and password and you are ready to go.
Before executing the script goto php.ini file and remove the ; that is infront of extension=php_openssl.dll
Then restart your server.
Leave a comment if you need any help.
Monday, May 11, 2009
check only for numbers in JavaScript
This code will show you how to check only for numbers in JavaScript.
<script type="text/javascript">
var input = '112334';
var vali_regex = /^[0-9]+$/;
if(input.match(vali_regex))
{
alert('This is a number');
}
else
{
alert('Not a number');
}
</script>
Monday, May 4, 2009
How to search an array in php
You can use array_search() function to easily search values in an php array. If you didn't know about this function you should have probarbly used a foreach or for loop to find a matching value in your array.
array_search() will return the array key if found the given value. This function is case sensitive to strings.
array_search() will return the array key if found the given value. This function is case sensitive to strings.
<?php
$my_array = array('1', 'kuppiya', 'Tom', 'Hello');
$key = array_search('Tom', $my_array);
if($key === false)
{
echo 'Not found';
}
else
{
echo $my_array[$key];
}
?>
Thursday, April 30, 2009
Alternative to classic LightBox - Sexy LightBox 2.1
Sexy LightBox 2.1 is a lightweight and elegant replacement for classic Lightboxes you use. Comes with a MIT license and very easy to integrate.
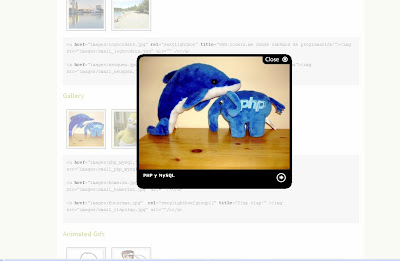
http://www.coders.me/ejemplos/sexy-lightbox-2/
http://www.coders.me/ejemplos/sexy-lightbox-2/
Tuesday, April 28, 2009
How to check upload file extension (JavaScript)
Before uploading a file it's a good idea to check for valid file extensions you want.
Even though you can easily bypass JavaScript validation by disabling JavaScript in the browser, you can prevent genuine user errors by implementing this code.
var valid_extensions = /(.jpg|.jpeg|.gif)$/i;
Check for .jpg, .jpeg and .gif file extentions in the end of the string
| - OR
i - Case Insensitive
$ - END
Even though you can easily bypass JavaScript validation by disabling JavaScript in the browser, you can prevent genuine user errors by implementing this code.
<html>
<head>
<title>Upload Check</title>
<script type="text/javascript">
function vali_type()
{
var id_value = document.getElementById('up').value;
if(id_value != '')
{
var valid_extensions = /(.jpg|.jpeg|.gif)$/i;
if(valid_extensions.test(id_value))
{
alert('OK');
}
else
{
alert('Invalid File')
}
}
}
</script>
</head>
<body>
<input type="file" name="up" id="up"
onBlur="vali_type()" />
</body>
</html>
var valid_extensions = /(.jpg|.jpeg|.gif)$/i;
Check for .jpg, .jpeg and .gif file extentions in the end of the string
| - OR
i - Case Insensitive
$ - END
Monday, April 27, 2009
mysql_real_escape_string() is not working properly
Is the function mysql_real_escape_string() return a empty value for you? or does it not work properly as you expected.
The most common mistake is the use of the mysql_real_escape_string() before a database connection.
Make sure you use the function after a successful database connection.
If E_WARNING error level is enabled in your error_reporting you will get a E_WARNING for this mistake. It's always a good idea to make error_reporting E_ALL in the development cycle.
http://www.php.net/mysql_real_escape_string
Read the Notes section for more details.
The most common mistake is the use of the mysql_real_escape_string() before a database connection.
Make sure you use the function after a successful database connection.
If E_WARNING error level is enabled in your error_reporting you will get a E_WARNING for this mistake. It's always a good idea to make error_reporting E_ALL in the development cycle.
http://www.php.net/mysql_real_escape_string
Read the Notes section for more details.
Saturday, April 11, 2009
List of Browser User Agents to use with php curl
Here is a simple list of user agents that can be used with php curl or JavaScript
(In windows)
IE 7 - Mozilla/4.0 (compatible; MSIE 7.0; Windows NT 5.1; SU 3.21; .NET CLR 2.0.50727; .NET CLR 3.0.04506.648; .NET CLR 3.5.21022)
Chrome - Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US) AppleWebKit/525.19 (KHTML, like Gecko) Chrome/1.0.154.53 Safari/525.19
Firefox 3.0 Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.9.0.8) Gecko/2009032609 Firefox/3.0.8
Firefox 2.0 - Mozilla/5.0 (Windows; U; Windows NT 5.1; en-GB; rv:1.8.1.6) Gecko/20070725 Firefox/2.0.0.6
Safari - Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US) AppleWebKit/525.28 (KHTML, like Gecko) Version/3.2.2 Safari/525.28.1
Opera - Opera/10.00 (Windows NT 5.1; U; en) Presto/2.2.0
(In windows)
IE 7 - Mozilla/4.0 (compatible; MSIE 7.0; Windows NT 5.1; SU 3.21; .NET CLR 2.0.50727; .NET CLR 3.0.04506.648; .NET CLR 3.5.21022)
Chrome - Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US) AppleWebKit/525.19 (KHTML, like Gecko) Chrome/1.0.154.53 Safari/525.19
Firefox 3.0 Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.9.0.8) Gecko/2009032609 Firefox/3.0.8
Firefox 2.0 - Mozilla/5.0 (Windows; U; Windows NT 5.1; en-GB; rv:1.8.1.6) Gecko/20070725 Firefox/2.0.0.6
Safari - Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US) AppleWebKit/525.28 (KHTML, like Gecko) Version/3.2.2 Safari/525.28.1
Opera - Opera/10.00 (Windows NT 5.1; U; en) Presto/2.2.0
Sunday, April 5, 2009
Wedding of two SLIIT Batchmates
Wednesday, April 1, 2009
Get php time diff (time diffence)
Following code will show you how to get time difference in second from a two datetime values. Remember datetime should be in this format: yyyy-mm-dd hr:min:sec
$dbdate_seconds = strtotime($dbdate);
$inputdate_seconds = strtotime($inputdate);
$time_diff = $dbdate_seconds - $inputdate_seconds;
//you will get the time difference in seconds, divide by 60 to make it minutes
or you can use the mysql TIMEDIFF
$query = select TIMEDIFF(field_name, '$input_date') from tbl_name where condition
$dbdate_seconds = strtotime($dbdate);
$inputdate_seconds = strtotime($inputdate);
$time_diff = $dbdate_seconds - $inputdate_seconds;
//you will get the time difference in seconds, divide by 60 to make it minutes
or you can use the mysql TIMEDIFF
$query = select TIMEDIFF(field_name, '$input_date') from tbl_name where condition
Saturday, March 28, 2009
Expresso Best Way to Learn Regular Expression
Expresso is a free tool where you can master the art of writing complex regular expressions in no time. You also got a very good tutorial within the software to get started on regular expressions.
It also contain regular expression library to assist you in common problems.
http://www.ultrapico.com/Expresso.htm
It also contain regular expression library to assist you in common problems.
http://www.ultrapico.com/Expresso.htm
Wednesday, March 25, 2009
Notice: Undefined index: (php)
Notice: Undefined index:
Sometimes you get this notice (This is not a error). lets see a example why you get this notice or warning.
When you initially load this page you will get a notice like following.
.PNG)
This notice is shown because your php.ini file has enabled Notices to display or you may have a set error_reporting() function to E_ALL or to display notices.
The reason for the notice because when the page is load it search for the $_POST["name"] and $_POST["number"] which actually not available, $_POST indexes are set when you submit the form not when you just load the page.
Solutions:
1) you can fix the error reporting levels in php.ini or by error_reporting() function.
or
Change the code like this.
This will make the code run only when you submit the add button.
Sometimes you get this notice (This is not a error). lets see a example why you get this notice or warning.
<?php
error_reporting(E_ALL);
echo $name= $_POST["name"];
echo $number= $_POST["number"];
?>
<html>
<body>
<form name="form1" method="POST" action="">
<table border="0">
<tr>
<td>Name :</td>
<td> <input type="text" name="name" /></td>
</tr>
<tr>
<td>Tel: Number :</td>
<td> <input type="text" name="number" /></td>
</tr>
<tr>
<td> </td>
<td><input type="submit" name="sub" value="Add" /></td>
</tr>
</table>
</form>
</body>
</html>
When you initially load this page you will get a notice like following.
This notice is shown because your php.ini file has enabled Notices to display or you may have a set error_reporting() function to E_ALL or to display notices.
The reason for the notice because when the page is load it search for the $_POST["name"] and $_POST["number"] which actually not available, $_POST indexes are set when you submit the form not when you just load the page.
Solutions:
1) you can fix the error reporting levels in php.ini or by error_reporting() function.
or
Change the code like this.
if(isset($_POST['sub']))
{
echo $name= $_POST["name"];
echo $number= $_POST["number"];
}
This will make the code run only when you submit the add button.
Sunday, March 22, 2009
How to change Mysql database storage engine
The default Mysql database storage engine is MyISAM. But if you want to implement foreign key constraints you should have the InnoDB storage engine. Lets see how we can change it. I will use the phpMyAdmin to do the operation.
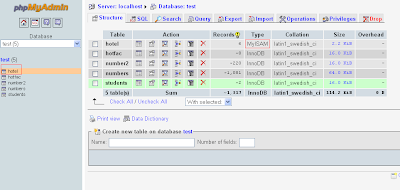
Select the table you want and press the "Operations" tab.
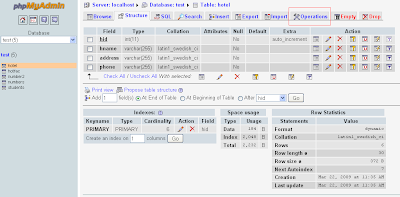
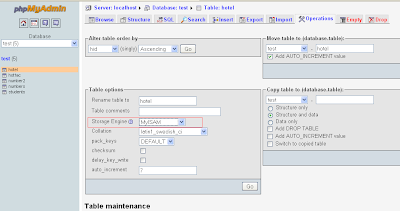
Now select a Storage Engine you like from the drop down menu and press "Go" button.
Select the table you want and press the "Operations" tab.
Now select a Storage Engine you like from the drop down menu and press "Go" button.
Thursday, March 19, 2009
Find JavaScript Errors Easily
Monday, March 16, 2009
ServerSide JavaScript will be popular like php
In the near future ServerSide JavaScript (SSJS) will be popular like php. Eventhough ServerSide JavaScript (SSJS) has been around for sometime it never got wide publicity. Been a object oriented and a simple scripting language in nature it will be pretty easy to write dynamic code using (SSJS). I first heard about ServerSide JavaScript (SSJS) from Aptana Jaxer.
You can find a list of ServerSide JavaScript (SSJS) uses from this wiki article.
http://en.wikipedia.org/wiki/Server-side_JavaScript
You can find a list of ServerSide JavaScript (SSJS) uses from this wiki article.
http://en.wikipedia.org/wiki/Server-side_JavaScript
Saturday, March 14, 2009
How to integrate a web editor (Rich Text Editor)
Lets see how to integrate TinyMCE into a web page
Goto http://tinymce.moxiecode.com and download the TinyMCE, select the main package to download.

Now click on the example tab

Now you can see the full featured example. You can find more example links also.
Click on the View Source.

Click on the view source.

Now copy and paste the code to your html file.
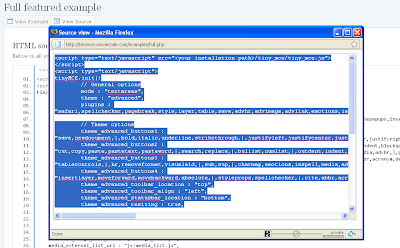
Remember to correctly change the installation path

Now you are done, Experiment with other examples also. If you need any help you can get my email from the profile.
Goto http://tinymce.moxiecode.com and download the TinyMCE, select the main package to download.
Now click on the example tab
Now you can see the full featured example. You can find more example links also.
Click on the View Source.
Click on the view source.
Now copy and paste the code to your html file.
Remember to correctly change the installation path
Now you are done, Experiment with other examples also. If you need any help you can get my email from the profile.
Thursday, March 12, 2009
How to use MD5 and SHA1 in php (cryptography)
MD5 - Message-Digest algorithm 5
SHA1 - Secure Hash Algorithm
These are the two main inbuilt functions used in php to encrypt sensitive data.
SHA1 is now consider and recommended to be more secure than MD5.
If you run above code your will see the encrypted string of the word password.
You can clearly see that sha1 is longer than md5. sha1 is encrypted with more bits.
Once a value is encrypted you cannot reaverse the process. So how you gonna check the values again? check the following code.
Always remember to encrypt the input data with relevant encryption method before comparing values.
SHA1 - Secure Hash Algorithm
These are the two main inbuilt functions used in php to encrypt sensitive data.
SHA1 is now consider and recommended to be more secure than MD5.
<?php
echo md5('password'); //5f4dcc3b5aa765d61d8327deb882cf99
echo '<br /><br />';
echo sha1('password'); //5baa61e4c9b93f3f0682250b6cf8331b7ee68fd8
?>
If you run above code your will see the encrypted string of the word password.
You can clearly see that sha1 is longer than md5. sha1 is encrypted with more bits.
Once a value is encrypted you cannot reaverse the process. So how you gonna check the values again? check the following code.
<?php
$stored_password = sha1('my_pass');
$pass = $_POST['pass'] // input password
if($stored_password == sha1($pass))
{
echo 'correct password';
}
else
{
echo 'wrong password';
}
?>
Always remember to encrypt the input data with relevant encryption method before comparing values.
Tuesday, March 10, 2009
How to make the site feed available for browsers
IE RSS feed browser icon

Firefox RSS feed browser icon

You can easily make available your feed to IE or Firefox by adding one simple line of code.
Add this line right after tag.
Thats it now your feed is available automatically by Firefox and IE, This will also help feed aggregator automatically find and index your feed.
Firefox RSS feed browser icon
You can easily make available your feed to IE or Firefox by adding one simple line of code.
Add this line right after tag.
<link rel="alternate" type="application/rss+xml" title="siteName.com RSS News Feed" href="feed path" />
Thats it now your feed is available automatically by Firefox and IE, This will also help feed aggregator automatically find and index your feed.
Wednesday, March 4, 2009
How to create a popup DatePicker for a text box using jQuery
For this we can use a very nice widget created by jQuery.
First we will download the datepicker widget from the site
http://jqueryui.com/download
Only select the Datepicker widget to download
After downloading the zip file, extract the files into a folder called jquery.
You can read more about this Datepicker from here.
http://jqueryui.com/demos/datepicker/
First we will download the datepicker widget from the site
http://jqueryui.com/download
Only select the Datepicker widget to download
After downloading the zip file, extract the files into a folder called jquery.
<html>
<head>
<title>jQuery UI Datepicker - Default functionality</title>
<link type="text/css" href="jquery/theme/ui.all.css" rel="Stylesheet" />
<script type="text/javascript" src="jquery/jquery-1.3.1.js"></script>
<script type="text/javascript" src="jquery/jquery-ui-personalized-1.6rc6.js"></script>
<script type="text/javascript">
$(function() {
$("#datepicker").datepicker();
});
</script>
</head>
<body>
<p>Date: <input type="text" id="datepicker"></p>
</body>
</html>
You can read more about this Datepicker from here.
http://jqueryui.com/demos/datepicker/
Saturday, February 28, 2009
How to check for a valid date using php
php have given the checkdate() function to validate user inputted dates.
checkdate($month, $day, $year) all parameters must be integer (numbers).
checkdate($month, $day, $year) all parameters must be integer (numbers).
<?php
$month = $input_month; // should be a number
$day = $input_day; // should be a number
$year = $input_year; // should be a number
if(checkdate($month, $day, $year))
{
echo 'Valid';
}
else
{
echo 'Not valid';
}
?>
Sunday, February 22, 2009
Get width and height of a swf / flash file using php
You can use getimagesize() function to get width and height of a swf /flash file.
getimagesize() is also used to get size of images.
To find out supported formats and return values go to this link
http://www.php.net/getimagesize
Following code is a simple example on how to get the width and height of a swf file.
getimagesize() is also used to get size of images.
To find out supported formats and return values go to this link
http://www.php.net/getimagesize
Following code is a simple example on how to get the width and height of a swf file.
list($width, $height) = getimagesize("file_name.swf");
Sunday, February 15, 2009
Right date format to store in mysql - YYYY-MM-DD HH:MM:SS
When using the php date function you can format the date in lot of ways.
But when you store date and time in mysql it is really convenient to store date in the mysql friendly format YYYY-MM-DD HH:MM:SS
If you use the above format you can easily use mysql date functions to manipulate and do calculation on stored dates without using php functions.
Example functions
DATEDIFF() - Subtract two dates
ADDDATE() - Add dates
http://dev.mysql.com/doc/refman/5.1/en/date-and-time-functions.html
But when you store date and time in mysql it is really convenient to store date in the mysql friendly format YYYY-MM-DD HH:MM:SS
If you use the above format you can easily use mysql date functions to manipulate and do calculation on stored dates without using php functions.
Example functions
DATEDIFF() - Subtract two dates
ADDDATE() - Add dates
http://dev.mysql.com/doc/refman/5.1/en/date-and-time-functions.html
Tuesday, February 10, 2009
How to create foreign key in mysql (InnoDB)
Yes you can definitely create foreign key constraints on mysql. but it can only be enforced in InnoDB storage engine. The default storage engine is MyISAM, you cannot create foreign key constraints in MyISAM. So make sure you create your tables using InnoDB storage engine.
If you are using a phpMyAdmin to work with mysql, look at the following ScreenShot.

Remember, before making a column available for referencing you should make sure that child column has a Index.


Now using Relation view link you can easily create foreign key constraints

If you are using a phpMyAdmin to work with mysql, look at the following ScreenShot.
Remember, before making a column available for referencing you should make sure that child column has a Index.
Now using Relation view link you can easily create foreign key constraints
Thursday, February 5, 2009
How to redirect a page in Dropdown (select) onchage
Sometimes you need to redirect or refresh a page when you select a new option in a dropdown box (select box) with some url parameters (query string).
Look at following code and try it yourself.
Look at following code and try it yourself.
<html>
<head>
<title>DropDown Redirect OnChange</title>
<script type="text/javascript">
function chng_page()
{
/*
get the selected option index
it start with 0
*/
var select_index = document.getElementById('names').selectedIndex;
// use alert(select_index); see the value
//get the value of selected option
var option_value = document.getElementById('names').options[select_index].value;
//redirect with value as a url parameter
window.location = 'page.php?id=' + option_value;
}
</script>
</head>
<body>
we are using onchange event to
call a javascript function
<br />
<select name="names" id="names" onchange="chng_page()">
<option value="0">select</option>
<option value="1">kuppiya.com</option>
<option value="2">kusal</option>
</select>
</body>
</html>
Sunday, February 1, 2009
How to add a html
tags to newline characters in php - nl2br()
The easiest way to add a HTML break
to all new lines in a string is to use the php nl2br() function. This is really great when you want to restore new lines from a user input by a textarea where user input could get into several lines.
to all new lines in a string is to use the php nl2br() function. This is really great when you want to restore new lines from a user input by a textarea where user input could get into several lines.
<?php
echo nl2br("line1\n line2");
?>
Wednesday, January 28, 2009
How to find current shell in Ubuntu / Linux
Saturday, January 24, 2009
How to put text content around a image using DIV
![]() These are some sample text to show you how to put content like this around the images. This technique is useful when you are writing articles with images. You should remember to set vspace and hspace properties in the img tag to correctly format the content. Look at the following code try it your self |
<table border="0" align="center" width="375">
<tr><td>
<div id="image"><img src="http://kuppiya.com/toplogo2.jpg"
vspace="3" align="left" border="0" hspace="3" /></div>
<div>These are some sample text to show you how to put content
like this around the images. This technique is useful when you are
writing articles with images. You should remember to set
vspace and hspace properties in the img tag to correctly format the
content. Look at the following code try it your self </div>
</td></tr>
</table>
Tuesday, January 20, 2009
How to merge two arrays together in php
When you need to join or merge two arrays together you can use the php array_merge() function to do it. This function will return the merged array.
Look at the following code and see how the index reassigned.
Look at the following code and see how the index reassigned.
<?php
$first_array = array(1, 2, 3, 4, 5, 6);
$second_array = array(10, 9, 7, 3, 5);
$new_array = array_merge($first_array, $second_array);
echo '<pre>';
print_r($new_array);
echo '</pre>';
/*
OUTPUT
Array
(
[0] => 1
[1] => 2
[2] => 3
[3] => 4
[4] => 5
[5] => 6
[6] => 10
[7] => 9
[8] => 7
[9] => 3
[10] => 5
)
*/
?>
Thursday, January 15, 2009
Remove duplicate values from an array in php - array_unique
You can use array_unique() function get unique values out of a long array.
function will return an array with unique values and will preserve the keys.
function will return an array with unique values and will preserve the keys.
<?php
$my_array = array(1, 2, 3, 3, 4, 4, 1, 5, 6, 6, 7);
$my_unique_array = array_unique($my_array);
echo '<pre>';
print_r($my_unique_array);
echo '</pre>';
/*
OUTPUT:
Array
(
[0] => 1
[1] => 2
[2] => 3
[4] => 4
[7] => 5
[8] => 6
[10] => 7
)
*/
?>
Sunday, January 11, 2009
How to put a value to the end of an array - array_push()
You can use array_push() function to pass a value to the end of an array.
array_push(current_array , value )
array_push() accept two basic parameters, first one is the current array you want to push the value. after that you can pass any number of values separated by commas.
array_push(current_array , value )
array_push() accept two basic parameters, first one is the current array you want to push the value. after that you can pass any number of values separated by commas.
<?php
$my_array = array('Me', 'You');
array_push($my_array, 'Others');
print_r($my_array);
/*
Array
(
[0] => Me
[1] => You
[2] => Others
)
*/
?>
Wednesday, January 7, 2009
How to find a specific file type inside a folder using php - glob()
Using the glob() function you can search only for a given file type inside a folder.
glob() will return an array containg all file names with the given file type.
Following code will show you how to get a list of only .gif image files from the image folder.
glob() will return an array containg all file names with the given file type.
Following code will show you how to get a list of only .gif image files from the image folder.
<?php
//get only .gif files from images folder
$files_list = glob("images/*.gif");
foreach($files_list as $files)
{
echo $files;
echo '<br />';
}
?>
Thursday, January 1, 2009
How to remove an element from an array variable in php
php unset() will not only unset a single variable, you can also remove elements from a array variable using unset(). Look at the following code.
See that the index 2 is not in the array any more and the $my_array is not in the correct index sequence. you can reassign indexes using the array_values()
See that the index 2 is not in the array any more and the $my_array is not in the correct index sequence. you can reassign indexes using the array_values()
<?php
$my_array = array('Hello', 'Bye', 'Kusal', 'kuppiya');
unset($my_array[2]); // remove kusal from array
print_r($my_array);
/*
Result:
Array ( [0] => Hello [1] => Bye [3] => kuppiya )
*/
?>

Subscribe to:
Posts (Atom)